Types¶
This section describes the object model for simple feature geometry and classs to create geometry features from Lua objects.
SQL/MM | Lua |
---|---|
ST.Point() |
gis.Point |
ST.LineString() |
gis.LineString |
ST.LinearRing() |
gis.LinearRing |
ST.Polygon() |
gis.Polygon |
ST.GeometryCollection() |
gis.GeometryCollection |
ST.MultiPoint() |
gis.MultiPoint |
ST.MultiLineString() |
gis.MultiLineString |
ST.MultiPolygon() |
gis.MultiPolygon |
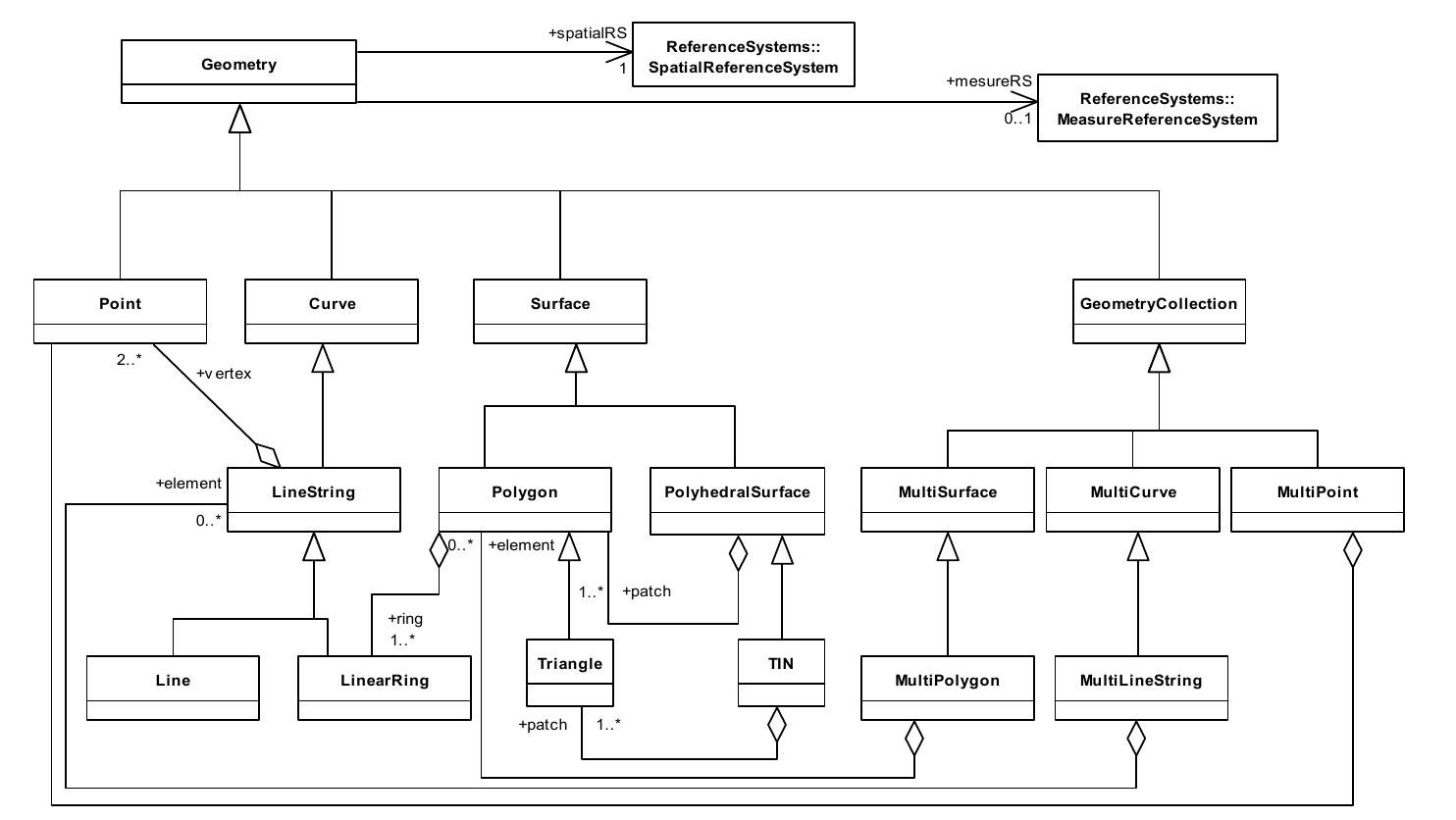
-
class
gis.
Geometry
¶ Geometry is the root class of the hierarchy. Geometry is an abstract (non-instantiable) class.
-
class
gis.
Point
({lon, lat}, srid)¶ -
class
gis.
Point
({x, y}, srid) -
class
gis.
Point
({x, y, z}, srid) Parameters: - x (number) – x
- y (number) – y
- z (number) – z
- srid (uint) – Spatial Reference System Identifier
Returns: point
Return type: Raises: on error
A Point is a 0-dimensional
geometric
object and represents a single location in coordinate space.tarantool> gis.Point({37.17284, 55.74495}, 4326) --- - POINT (37.17284 55.74495) ... tarantool> gis.Point({2867223.8779605, 2174199.925114, 5248510.4102534}, 4328) --- - POINT Z (2867223.8779605 2174199.925114 5248510.4102534) ...
-
class
gis.
Curve
¶ A Curve is a 1-dimensional
geometric
object usually stored as a sequence ofPoints
, with the subtype of Curve specifying the form of the interpolation between Points. ISO 19123 standard defines only one subclass of Curve,LineString
, which uses linear interpolation between Points. Curve is an abstract (non-instantiable) class.
-
class
gis.
LineString
({{lon, lat}, point, {lon, lat}, ...}, srid)¶ -
class
gis.
LineString
({{x, y}, point, {x, y}, ...}, srid) -
class
gis.
LineString
({{x, y, z}, point, {x, y}, ...}, srid) -
class
gis.
LineString
(linestring, srid) -
class
gis.
LineString
(linearring, srid) Parameters: - x (double) – x
- y (double) – y
- z (double) – z
- point (Point) – point
- linestring (LineString) – linestring
- linearring (LinearRing) – linearring
- srid (integer) – Spatial Reference System Identifier
Returns: linestring
Return type: A LineString is a
Curve
with linear interpolation betweenPoints
. Each consecutive pair of Points defines aLine
segment.tarantool> gis.LineString({{37.279357, 55.849493}, {37.275152, 55.865005}, gis.Point({37.261676, 55.864041}, 4326)}, 4326) --- - LINESTRING (37.279357 55.849493, 37.275152 55.865005, 37.261676 55.864041) ...
tarantool> gis.LineString({{2855517, 2173695, 5255053}, {2854539, 2172620, 5256022}, {2855120, 2172002, 5255962}}, 4328) --- - LINESTRING Z (2855517 2173695 5255053, 2854539 2172620 5256022, 2855120 2172002 5255962) ...
-
class
gis.
LinearRing
({{lon, lat}, point, {lon, lat}, ...}, srid)¶ -
class
gis.
LinearRing
({{x, y}, point, {x, y}, ...}, srid) -
class
gis.
LinearRing
({{x, y, z}, point, {x, y}, ...}, srid) -
class
gis.
LinearRing
(linestring, srid) -
class
gis.
LinearRing
(linearring, srid) Parameters: - x (double) – x
- y (double) – y
- z (double) – z
- point (Point) – point
- linestring (LineString) – linestring
- linearring (LinearRing) – linearring
- srid (integer) – Spatial Reference System Identifier
Returns: linearring
Return type: A LinearRing is a
LineString
that is both closed and simple.tarantool> gis.LinearRing({{37.279357, 55.849493}, {37.275152, 55.865005}, {37.261676, 55.864041}, {37.279357, 55.849493}}, 4326) --- - LINEARRING (37.279357 55.849493, 37.275152 55.865005, 37.261676 55.864041, 37.279357 55.849493) ...
-
class
gis.
Line
¶ A Line is a
LineString
with exactly 2Points
.Tarantool/GIS doesn’t provide this subclass, please use
LineString
instead.
-
class
gis.
Surface
¶ A Surface is a 2-dimensional
geometric
object. A simple Surface may consists of a single “patch” that is associated with one “exterior boundary” and 0 or more “interior” boundaries. A single such Surface patch in 3-dimensional space is isometric to planar Surfaces, by a simple affine rotation matrix that rotates the patch onto the plane z = 0. If the patch is not vertical, the projection onto the same plane is an isomorphism, and can be represented as a linear transformation, i.e. an affine.
-
class
gis.
Polygon
({ {{x, y, z}, point, ...}, linearring, ...}, srid)¶ Parameters: - x (double) – x
- y (double) – y
- z (double) – z
- point (Point) – point
- linearring (LinearRing) – linearring
- srid (integer) – Spatial Reference System Identifier
Returns: Polygon
Return type: A Polygon is a planar
Surface
defined by 1 exterior boundary and 0 or more interior boundaries. Each interior boundary defines a hole in the Polygon. The exterior boundaryLinearRing
defines the “top” of the surface which is the side of the surface from which the exterior boundary appears to traverse the boundary in a counter clockwise direction. The interiorLinearRings
will have the opposite orientation, and appear as clockwise when viewed from the “top”.tarantool> shell = { > {37.279357, 55.849493}; > {37.275152, 55.865005}; > {37.261676, 55.864041}; > {37.279357, 55.849493}; > } --- ... tarantool> hole = { > {37.267856, 55.853781}; > {37.269401, 55.858502}; > {37.273864, 55.854937}; > {37.267856, 55.853781}; > } --- ... tarantool> gis.Polygon({shell, hole}, 4326) --- - POLYGON ((37.279357 55.849493, 37.275152 55.865005, 37.261676 55.864041, 37.279357 55.849493), (37.267856 55.853781, 37.269401 55.858502, 37.273864 55.854937, 37.267856 55.853781)) ...
-
class
gis.
Triangle
¶ A Triangle is a
Polygon
with 3 distinct, non-collinear vertices and no interior boundaries. Tarantool/GIS doesn’t provide this subclass, please usePolygon
instead.
-
class
gis.
PolyhedralSurface
¶ A PolyhedralSurface is a contiguous collection of polygons, which share common boundary segments. For each pair of polygons that “touch”, the common boundary shall be expressible as a finite collection of
LineStrings
. Each such :class`LineString` shall be part of the boundary of at most 2 Polygon patches.Tarantool/GIS currently doesn’t implement this feature.
-
class
gis.
TIN
¶ Inherits
Surface
A TIN (triangulated irregular network) is a
PolyhedralSurface
consisting only of Triangle patches.Tarantool/GIS currently doesn’t implement this feature.
-
class
gis.
GeometryCollection
({geometry, geometry, ...}, srid)¶ Parameters: - geometry (Geometry) – geometry
- srid (integer) – Spatial Reference System Identifier
Returns: collection
Return type: A GeometryCollection is a geometric object that is a collection of some number of geometric objects. All the elements in a GeometryCollection shall be in the same Spatial Reference System. This is also the Spatial Reference System for the GeometryCollection. GeometryCollection places no other constraints on its elements. Subclasses of GeometryCollection may restrict membership based on dimension and may also place other constraints on the degree of spatial overlap between elements.
tarantool> point = gis.Point({37.17284, 55.74495}, 4326) --- ... tarantool> linestring = gis.LineString({{37.275152, 55.865005}, {37.261676, 55.864041}}, 4326) --- ... tarantool> gis.GeometryCollection({point, linestring}, 4326) --- - GEOMETRYCOLLECTION (POINT (37.17284 55.74495), LINESTRING (37.275152 55.865005, 37.261676 55.864041)) ...
-
class
gis.
MultiPoint
({{lon, lat}, point, {lon, lat}, ...}, srid)¶ -
class
gis.
MultiPoint
({{x, y}, point, {x, y}, ...}, srid) -
class
gis.
MultiPoint
({{x, y, z}, point, {x, y}, ...}, srid) -
class
gis.
MultiPoint
(linestring, srid) -
class
gis.
MultiPoint
(linearring, srid) Parameters: - x (double) – x
- y (double) – y
- z (double) – z
- point (Point) – point
- linestring (LineString) – linestring
- linearring (LinearRing) – linearring
- srid (integer) – Spatial Reference System Identifier
Returns: multipoint
Return type: A MultiPoint is a 0-dimensional
GeometryCollection
. The elements of a MultiPoint are restricted toPoints
. The Points are not connected or ordered in any semantically important way. A MultiPoint is simple if no two Points in the MultiPoint are equal (have identical coordinate values in X and Y).The boundary of a MultiPoint is the empty set.tarantool> gis.MultiPoint({{37.279357, 55.849493}, {37.275152, 55.865005}}, 4326) --- - MULTIPOINT (37.279357 55.849493, 37.275152 55.865005) ...
-
class
gis.
MultiCurve
¶ A MultiCurve is a 1-dimensional
GeometryCollection
whose elements areCurves
. This class is an abstract.
-
class
gis.
MultiLineString
({ {{x, y, z}, point, ...}, linestring, linearring, ...}, srid)¶ Parameters: - x (double) – x
- y (double) – y
- z (double) – z
- point (Point) – point
- linestring (LineString) – linestring
- linearring (LinearRing) – linearring
- srid (integer) – Spatial Reference System Identifier
Returns: MultiLineString
Return type: A MultiLineString is a
MultiCurve
whose elements are LineStrings.tarantool> linestrings = { > { > {37.279357, 55.849493}; > {37.275152, 55.865005}; > {37.261676, 55.864041}; > }; > gis.LineString({ > {37.267856, 55.853781}; > {37.269401, 55.858502}; > {37.273864, 55.854937}; > }, 4326); > } --- ... tarantool> gis.MultiLineString(linestrings, 4326) --- - MULTILINESTRING ((37.279357 55.849493, 37.275152 55.865005, 37.261676 55.864041), (37.267856 55.853781, 37.269401 55.858502, 37.273864 55.854937)) ...
-
class
gis.
MultiSurface
¶ A MultiSurface is a 2-dimensional
GeometryCollection
whose elements areSurfaces
, all using coordinates from the same coordinate reference system. The geometric interiors of any two Surfaces in a MultiSurface may not intersect in the full coordinate system. The boundaries of any two coplanar elements in a MultiSurface may intersect, at most, at a finite number of Points. If they were to meet along a curve, they could be merged into a single surface.This class is an abstract in Tarantool/GIS.
-
class
gis.
MultiPolygon
({polygon, {{{x, y, z}, point, ...}, linearring, ...}, ... }, srid)¶ Parameters: - x (double) – x
- y (double) – y
- z (double) – z
- point (Point) – point
- linearring (LinearRing) – linearring
- polygon (Polygon) – polygon
- srid (integer) – Spatial Reference System Identifier
Returns: multipolygon
Return type: A MultiPolygon is a
MultiSurface
whose elements arePolygons
.tarantool> polygons = { > {{ > {37.279357, 55.849493}; > {37.275152, 55.865005}; > {37.261676, 55.864041}; > {37.279357, 55.849493}; > }}; > {{ > {37.267856, 55.853781}; > {37.269401, 55.858502}; > {37.273864, 55.854937}; > {37.267856, 55.853781}; > }}; > } --- ... tarantool> gis.MultiPolygon(polygons, 4326) --- - MULTIPOLYGON (((37.279357 55.849493, 37.275152 55.865005, 37.261676 55.864041, 37.279357 55.849493)), ((37.267856 55.853781, 37.269401 55.858502, 37.273864 55.854937, 37.267856 55.853781))) ...